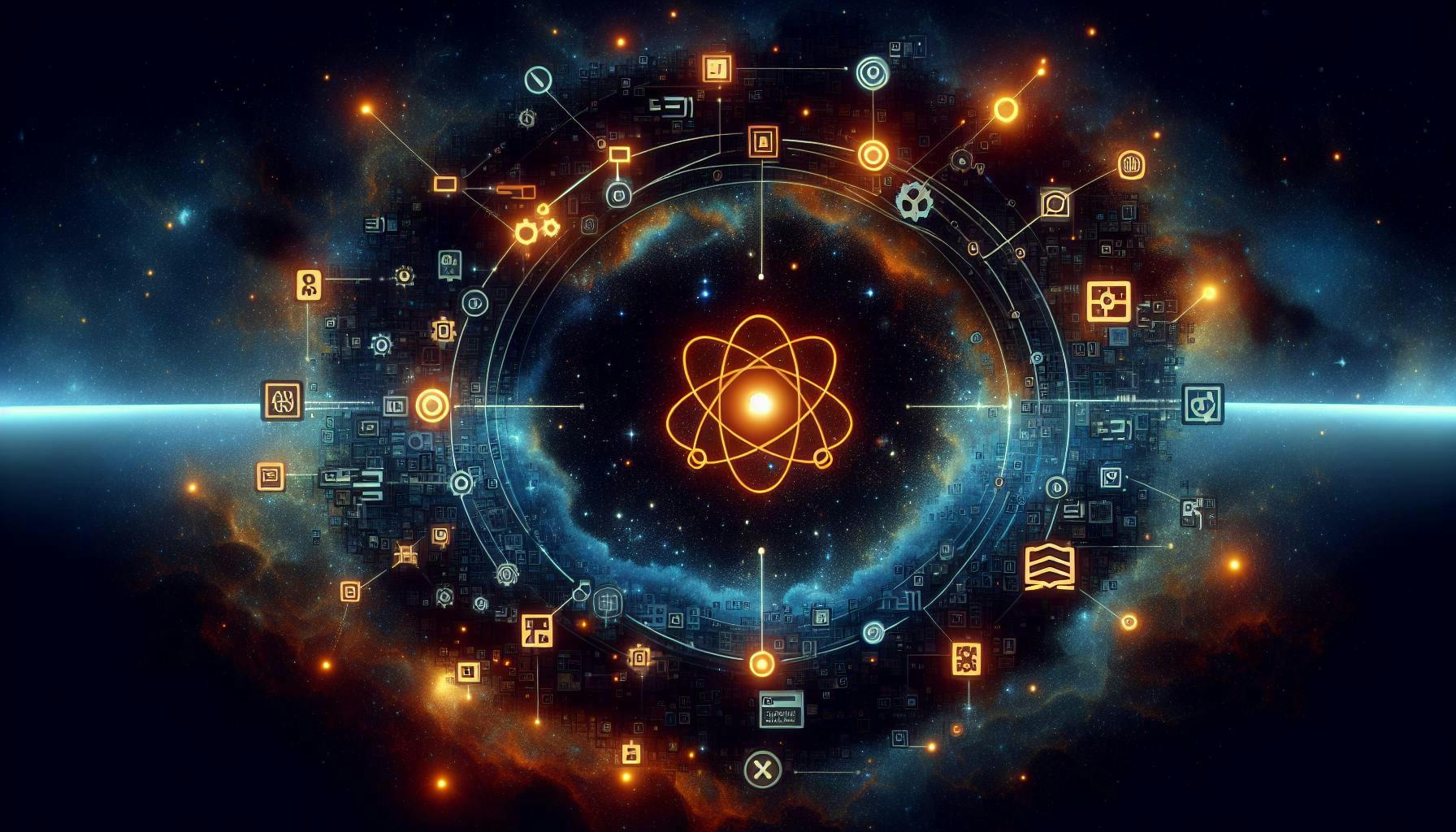
JSONPlaceholder API: The Easiest Fake REST API
Looking for an easy way to test your front-end application without the hassle of setting up a full backend? JSONPlaceholder API offers a simple solution with its fake REST API, delivering mock data for a variety of use cases. Here's what you need to know:
- Quick Start: Use basic GET requests to fetch fake data, such as posts, comments, and user profiles.
- Why It's Useful: It allows front-end developers to prototype and test without waiting for backend development.
- Key Features:
- Multiple endpoints for different data types (posts, comments, albums, etc.)
- Supports standard HTTP methods (GET, POST, PUT, PATCH, DELETE)
- Customizable responses and simulated latencies for more realistic testing
- Use Cases: Ideal for testing front-end code, creating API documentation, and setting up sandbox environments.
Compared to alternatives like Mocky.io and Mockable, JSONPlaceholder stands out for its ease of use and comprehensive features for front-end testing. Whether you're developing an app or learning about REST APIs, JSONPlaceholder provides valuable, realistic data without the complexity of a real backend.
Why Use a Mock REST API?
Using a fake REST API helps speed up making apps because the people working on the front part can do their job without needing the server part ready. They can use JSONPlaceholder to get realistic data to work with. This means they can start making things right away, without waiting.
Also, because the fake API gives consistent data, they can make sure everything works right. Later, when the real server is ready, they just need to switch where they are getting their data from.
Getting Started
To start using the JSONPlaceholder API, you can simply make a basic GET request. Here's a small piece of code to help you do that:
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json())
.then(json => console.log(json));
This code asks for data from the /posts
endpoint, which sends back a list of 100 pretend posts. The fetch
API gets a response back, and by using .json()
on this response, we turn the data into JSON format, which is then shown in the console.
First API Call
For your very first time calling the JSONPlaceholder API, trying the /posts
endpoint is a smart choice. It gives you a list of 100 pretend posts like this:
[
{
"userId": 1,
"id": 1,
"title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit",
"body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto"
},
// ...more posts
]
Each post has a unique id
, a userId
that shows who the pretend author is, a title
, and text in the body
. The information changes every time you ask for it, giving you different pretend posts to work with.
This lets you start making parts of your app or website that show posts, even if you don't have real data yet. JSONPlaceholder is a great tool for quickly testing out how your app looks and works with API data.
Features
JSONPlaceholder is a really easy-to-use fake REST API that's super helpful for developers when they're working on creating websites or apps without having a real backend ready yet.
Endpoints
JSONPlaceholder gives you access to a bunch of fake data through different paths:
/posts
- Gives you 100 fake blog posts/comments
- Gives you 500 fake comments/albums
- Gives you 100 fake albums/photos
- Gives you 5000 fake photos/todos
- Gives you 200 fake to-do items/users
- Gives you 10 fake user profiles
This fake data is perfect for testing how your website or app will look and work before the real data is available.
HTTP Methods
JSONPlaceholder lets you do everything you'd expect with a real API, including:
- GET - Get data from the server
- POST - Add new data
- PUT - Change data
- PATCH - Make partial changes to data
- DELETE - Remove data
This means you can fully test adding, changing, and removing data just like you would with a real server.
Customization Options
JSONPlaceholder also lets you tweak how it works to better fit your testing needs, like:
- Custom headers - Add special instructions for the server
- Simulated latency - Make the server respond more slowly to test how your app handles delays
- Status codes - Test how your app reacts to different server responses, like errors
- Query parameters - Use special commands in your request to filter, sort, or search the data
- Request body - Send data to the server when you're adding or changing things
- CORS - Make sure your app can talk to the server even if they're not on the same website
These features let you make the fake server behave more like your real server might, helping you catch problems early.
Use Cases
JSONPlaceholder API is super useful for a bunch of different situations when you're making apps:
Testing Front-End Code
The JSONPlaceholder API is a big help for checking your front-end code and how things work before you have real back-end APIs ready.
You can use the API to:
- Get and show fake data, like blog posts or user info
- Check forms for adding/updating stuff
- See how your app deals with errors when API calls don't work
This lets you build and test parts of your app without needing a real back-end first.
API Documentation
You can use JSONPlaceholder responses in API documentation to show example requests and responses.
For example, you could show what happens when you make a GET request to /posts
and what the fake JSON response looks like. This helps explain how your API works in a clear way using fake but realistic data.
Sandbox Environments
The API is also great for setting up a sandbox, or a safe space, to test things out.
Developers can try making different requests and see what kind of fake responses come back. This is good for testing apps that use API data without messing with real data.
This sandbox is great for trying things out, learning how an API works, or checking how your app handles unusual situations. Plus, you can reset it anytime you want.
sbb-itb-b2281d3
Alternatives
Let's look at how JSONPlaceholder stacks up against other tools like Mocky.io and Mockable that also let you create fake APIs.
Mocky.io
Mocky.io lets you make your own fake APIs with custom answers. Here's what it offers:
- A user-friendly way to build APIs by just dragging and dropping things
- You can pick what kind of responses you want, like how fast it should be or what it should say
- You can share your fake APIs with others and work on them as a team
- It shows your API's structure in a visual way
Compared to JSONPlaceholder, Mocky.io is more about visually creating fake endpoints quickly.
Mockable
Mockable is another tool for making simple fake APIs. It has some cool features:
- You can create fake APIs based on OpenAPI specs, which is a way to describe APIs
- There's a command line tool, so you can work with it directly from your terminal
- You can customize responses, like adding custom headers or making the server respond slower
- It lets you manage your team and share APIs
- You can see detailed reports on how your APIs are used
One big thing about Mockable is that it uses OpenAPI specs, so you can make fake APIs from your API descriptions. The command line tool also makes it easy to include these mock APIs in your automated workflows.
Conclusion
JSONPlaceholder API helps speed up making apps by letting you use fake data for testing. This means you can work on the look and feel of your app without needing the real data from the back end right away.
Key Takeaways
- Saves time - By using fake data, the people making the app and the ones setting up the server can work at the same time. This means the app can be ready faster.
- Flexible customization - With JSONPlaceholder, you can adjust things like how long it takes to get a response, what kind of errors to show, and more. This is great for making sure your app can handle different situations.
- Great docs and community - JSONPlaceholder has a lot of guides and a place where developers can talk and help each other out. This makes it easier to use and solve any issues.
In short, JSONPlaceholder makes it quicker to work on your app by letting you test with fake data. It gives you the freedom to try different things and get help when you need it, which means you can make a better app in less time.
Related Questions
How do I get a dummy API for testing?
If you need a fake API for testing, you can try using Mocki. It's a tool we made to help developers easily create and test APIs. With Mocki, you can visually set up your API, decide what the responses should be, and even add delays to see how your app handles slow responses. You can work together with your team by sharing your API setups and keeping track of how they're used. Mocki is great for making a custom mock API that fits exactly what you need for testing.
What is JSON placeholder API?
JSONPlaceholder is a free service that provides fake data for testing. You can use it for examples in documents, demos, or even for local testing. It gives you access to fake data for things like posts, comments, and photos. JSONPlaceholder supports all the usual web requests like GET, POST, and DELETE, and you can even customize the data by filtering, sorting, or searching. It's a useful tool for testing how your app works with data.
What is JSON data for developers?
JSON (JavaScript Object Notation) is a simple way to store and share data. Here's an example:
{
"name": "John",
"age": 30,
"isDeveloper": true
}
For developers, JSON makes it easy to handle data because it's straightforward and works well with JavaScript. It can represent basic data types like numbers, strings, and arrays. Since it's so simple, JSON is widely used for sending data between computers and for storing information.
Do rest APIs always use JSON?
No, REST APIs don't have to use JSON. They can send data in different formats like text, HTML, XML, or YAML. This flexibility lets REST APIs work with various types of data and clients. While JSON is popular, the ability to choose from different data formats means REST APIs can cater to many needs.