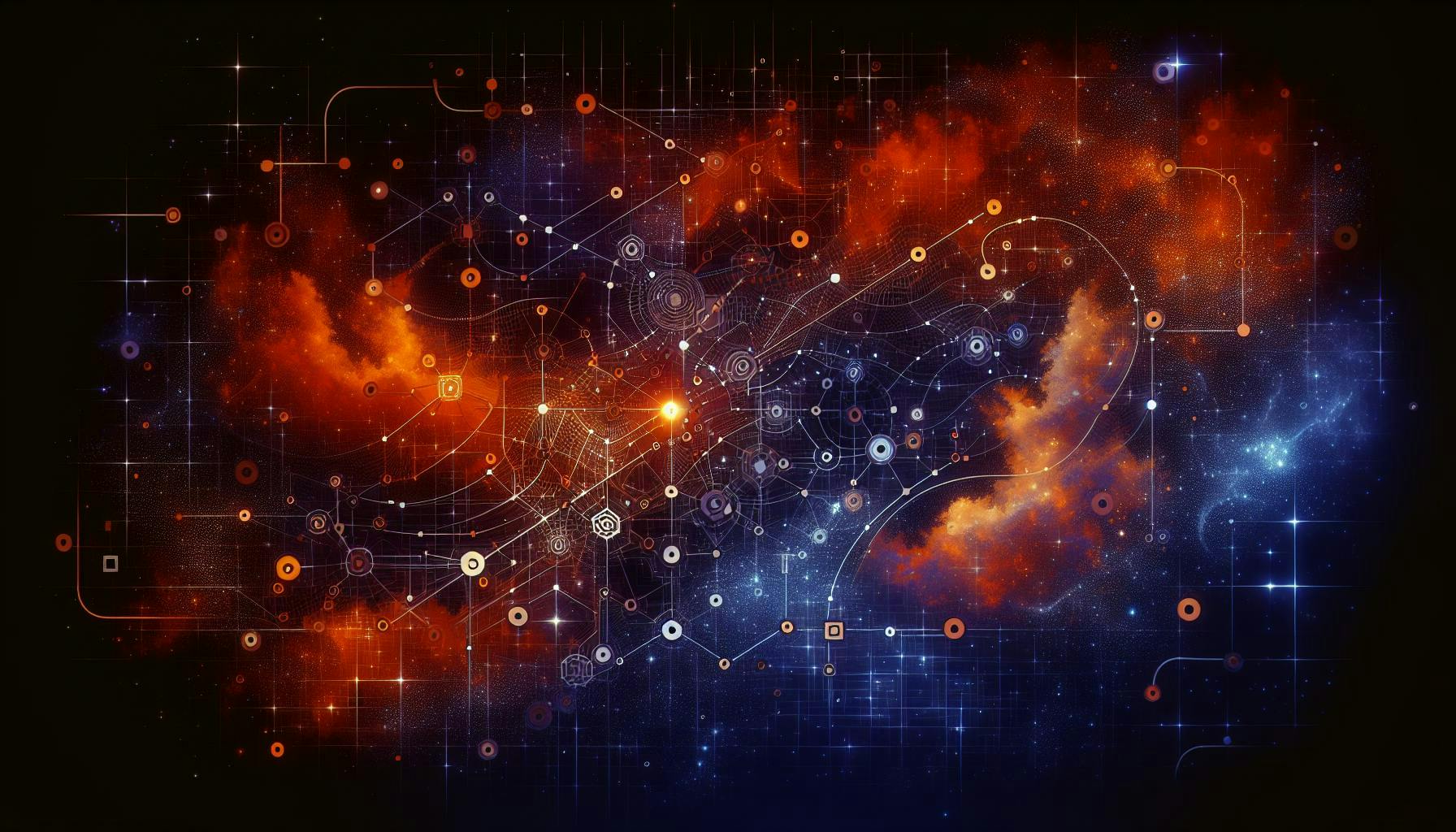
Get Started with JSONPlaceholder API
Getting started with the JSONPlaceholder API is a breeze for developers looking to mock data for testing and prototyping web projects. This free online tool offers fake but realistic data, ideal for front-end development without the backend hassle. Here's a quick guide to what you need to know:
- Why Use JSONPlaceholder? Rapid prototyping, simplified development, and predictable testing.
- Setting Up Your Environment: Basic knowledge of REST APIs and tools like Postman or cURL is necessary.
- Making Your First API Call: Simple examples using JavaScript and Python to fetch data.
- Exploring CRUD Operations: How to create, read, update, and delete data using the API.
- Advanced Features: From reading documentation and using async await in API calls to creating and deleting resources.
- Integrating JSONPlaceholder: Tips on persisting data, mocking login, seeding initial data, and swapping to real APIs.
- Alternative Tools and Comparisons: A look at how JSONPlaceholder compares to other tools like json-server, Mocky.io, Postman, Prism, and WireMock.
Whether you're a beginner or an experienced developer, JSONPlaceholder offers a straightforward way to speed up development and test your projects with ease.
Rapid Prototyping
With JSONPlaceholder, you can make up APIs to build and check your app's front end without needing a real backend. This means you can:
- Try out your app ideas quickly without waiting for the backend
- Focus on making the app user-friendly right away
- Learn from mistakes fast without much loss
Simplified Development
It cuts out the need to have a complex backend ready from the get-go, which lets you concentrate on crafting great user interfaces. Using this hosted API service means less work for developers.
- Work on the app's front end by itself
- Avoid getting stuck in too much detail too early
- Keep things simple at the start
Predictable Testing
This hosted API gives you a steady and reliable source of data for testing. Since the data format is set, your tests will be more stable.
- The same kind of data for all tests
- Fake logins and errors to check how your app handles them
- Do integration tests more smoothly
By offering ready-made fake data and APIs without the trouble of setting up real backends, JSONPlaceholder makes the app development process easier. This way, you can focus on the look and feel of your app while also making sure your tests run well.
Setting Up Your Environment
Before diving into JSONPlaceholder, make sure you have:
- A bit of knowledge about REST APIs and how to send requests over the internet
- A tool or a way to send these requests, like Postman, cURL, or even code in JavaScript or Python
- Access to the JSONPlaceholder API itself
That's really all you need. JSONPlaceholder is super straightforward and doesn't ask for any complex setup from your side.
Understanding REST APIs
REST stands for Representational State Transfer. It's a set of rules for making web services easy to use. Here’s what you need to remember:
- APIs let you request data through specific internet addresses called endpoints
- You'll use common web commands like GET, POST, PUT, PATCH, DELETE to ask for or send data
- The data you send or receive is usually in a format called JSON
With JSONPlaceholder, you're going to use these commands to ask for pretend data and see how it responds.
Using an API Client
To play around with JSONPlaceholder, you need a way to send your requests. Here are some tools that can help:
- Postman - A friendly app for making API requests
- cURL - A tool you use through the command line to send and receive data
- JavaScript - Great for making websites and front-end projects
- Python - Good for scripting and back-end programming
These tools help you specify what you're asking for, like which endpoint to hit and what kind of data you're dealing with.
Accessing the API
JSONPlaceholder is open for anyone to use for free, no account needed. And there's no limit on how much you can use it.
The main address for sending requests is:
https://jsonplaceholder.typicode.com
For example, if you want to get a list of posts, you would use:
https://jsonplaceholder.typicode.com/posts
And that’s pretty much it! Now you’re ready to start experimenting with the API using any HTTP client.
Making Your First API Call
Let's dive into how you can start using JSONPlaceholder to grab some data. We'll go through a simple example using JavaScript and Python to fetch a post.
JavaScript
Here's a basic way to get information on a specific post using JavaScript:
fetch('https://jsonplaceholder.typicode.com/posts/1')
.then(response => response.json())
.then(data => {
console.log(data)
})
Steps:
- We start with
fetch()
to ask for the post with ID 1. - Next, we make sure the answer we got is in a format we can easily work with, using
.json()
. - Finally, we show the post details using
console.log
.
That's all there is to it! You'll see the post's details as a neat package of information.
Python
To do the same thing in Python, here's what you do:
import requests
response = requests.get('https://jsonplaceholder.typicode.com/posts/1')
print(response.json())
Steps:
- First, we bring in the
requests
library so we can send web requests. - We use
requests.get()
to fetch the post. - We print out the post's details, which are neatly organized for us.
Just like that, you've made your first API call with Python, getting back some cool data on a post. Try grabbing different things like comments or albums to see how it works.
Exploring CRUD Operations
JSONPlaceholder lets you try out basic CRUD (Create, Read, Update, Delete) actions easily. Here's a simple guide to what each one does.
GET
To get data from JSONPlaceholder, we use the GET method. For example:
GET https://jsonplaceholder.typicode.com/posts
This gets a list of all posts.
GET https://jsonplaceholder.typicode.com/posts/1
This gets the details of the post with ID 1.
GET https://jsonplaceholder.typicode.com/posts?userId=7
This shows posts from user 7.
GET helps you:
- See all kinds of data like posts or comments
- Find specific items by ID
- Filter data using things like userId
It's mainly for reading data.
POST
To add something new, we use POST:
POST https://jsonplaceholder.typicode.com/posts
And include details like:
{
"title": "New Post",
"body": "This was created with POST",
"userId": 1
}
This makes a new post.
POST lets you:
- Add new stuff
- Give details in the request body
- Get back the new item with a new ID
It's for creating things.
PUT/PATCH
To change something that's already there, we use:
PUT https://jsonplaceholder.typicode.com/posts/1
With details like:
{
"id": 1,
"title": "Updated Post Title"
}
This changes the title of the post with ID 1.
Or:
PATCH https://jsonplaceholder.typicode.com/posts/1
With just the part you want to change:
{
"title": "Patched Title"
}
PUT changes everything, but PATCH just updates parts.
They're for editing stuff.
DELETE
To get rid of something, you can:
DELETE https://jsonplaceholder.typicode.com/posts/1
This removes the post with ID 1.
DELETE is used to:
- Take away data
- Test removing stuff
- But remember, it doesn't actually delete it from the server
It's for pretending to delete things.
By practicing these CRUD actions, you can see how apps manage data using JSONPlaceholder's fake API.
Advanced Features
After getting comfortable with the basics of JSONPlaceholder, you might wonder what else you can do with it. Let's look into some advanced features that can take your projects to the next level.
Reading Documentation
Understanding the documentation is key. It's like reading the manual before using a new gadget. The JSONPlaceholder documentation shows you all the different types of data you can work with and how to access them. It's a good idea to spend some time here to get familiar with what's possible.
Async Await for API Calls
When you make an API call, you're asking the server to send you some data. But this doesn't happen instantly. Using async await
in JavaScript makes handling these waits easier. It lets you write code that waits for the API call to finish before moving on, making your code cleaner and easier to understand.
Creating a Resource API Call
Creating new data on JSONPlaceholder is straightforward. For example, if you want to add a new post, you use a POST
request. This is similar to filling out a form on a website and hitting the submit button. The server then sends back a response, usually the new data you just created, including a new ID for it.
Delete Resource API Call
Just like adding new data, you can also remove it with a DELETE
request. Imagine there's a delete button next to every post on a website. When you click it, it sends a DELETE
request to the server. The server then responds, usually confirming that the post has been removed. Keep in mind, in JSONPlaceholder, the data isn't actually deleted but it simulates the process.
Launch JSON Server
For those who want to go even further, you can set up your own JSON server. This involves creating a db.json
file with your data and launching a server that uses this file. It's a bit like setting up your own version of JSONPlaceholder on your computer. This is great for testing and development purposes.
By exploring these advanced features, you'll get a better understanding of how APIs work and how you can use them in your projects. Whether it's reading the documentation, managing async operations, or even setting up your own server, there's a lot to learn and experiment with.
sbb-itb-b2281d3
Integrating JSONPlaceholder
Using JSONPlaceholder in your projects can make building and testing your apps faster. Here’s how you can include it in your work.
Persisting Data
Remember, JSONPlaceholder doesn’t keep data saved over time. But, you might want to keep some data around to act like it does. You could:
- Keep data in the browser using
localStorage
so it stays even when you refresh the page - Save data to a file on your computer
- Start your app with some preset data from a file
This helps your app remember things for testing, even though JSONPlaceholder doesn’t save anything for real.
Mocking Login
JSONPlaceholder doesn’t deal with logins. But you can pretend to by using fake user IDs or tokens.
For instance:
function login(username, password) {
// Pretend to check with API
if(username === 'foo' && password === 'bar') {
return generateUserToken()
} else {
return null
}
}
function makeApiCall(token) {
if(token) {
// Pretend to attach token to request
return fetchData()
} else {
throw Error('Unauthorized')
}
}
This way, you can try out parts of your app that need a user to be logged in without setting up a real login system.
Seeding Initial Data
When you first open your app, you might want it to already have some data, so it doesn’t look empty.
You could:
- Call an API to load data when the app starts
- Use a JSON file filled with starter data
- Put default data in localStorage
This makes your app seem more complete from the beginning.
Swapping to Real APIs
Once you’re ready for your real APIs, switching from JSONPlaceholder is easy.
For example:
// Starting with JSONPlaceholder
let API_ROOT = 'https://jsonplaceholder.typicode.com'
// Moving to your real API
API_ROOT = 'https://api.myapp.com'
Just by changing the URL, all your API calls will go to your real server, making the move smoother.
By following these steps, you can get a lot of testing done without needing everything built first.
Alternative Tools and Comparisons
When it comes to making fake APIs and getting dummy data for testing, JSONPlaceholder is super helpful. But, there are other tools out there too. Each has its own good and not-so-good points depending on what you need.
Here's how JSONPlaceholder stacks up against some other options:
Tool | Use Case | Ease of Setup | Customization Level |
---|---|---|---|
JSONPlaceholder | Quick prototyping | High | Low |
json-server | Custom mock APIs | Medium | High |
Mocky.io | Simple mocking | High | Medium |
Postman | Full API testing | Medium | High |
Prism | GraphQL mocking | Low | High |
WireMock | Stubbing | Low | High |
JSONPlaceholder
JSONPlaceholder is super easy to start with. You just hit its API endpoints to grab some fake data. But, it's pretty basic and doesn't let you change much.
json-server
With json-server, you can create your own fake REST API using a JSON file as your database. It's a bit more work to get going than JSONPlaceholder, but you can tweak it more to fit your needs.
Mocky.io
Mocky.io is kind of like JSONPlaceholder, but for mocking AJAX requests. It's almost as simple to use and gives you more options to make your fake endpoints just right.
Postman
Postman is a tool for deeper API testing. It's got a learning curve but can do a lot, like mock servers and check how your API works under different conditions.
Prism
If you're working with GraphQL, Prism is made for that. It helps you create detailed fake APIs and gives you a lot of control.
WireMock
WireMock is for making mock HTTP-based APIs by setting up fake responses. It needs Java and some coding but is very flexible.
So, JSONPlaceholder is awesome for quick and easy REST API mocks. But if you need more control or have specific needs, like working with GraphQL, other tools like json-server, Mocky.io, Postman, or Prism might be better.
Conclusion
JSONPlaceholder is a super helpful tool for anyone making websites and needs to test things out without having a full server setup. It's like a shortcut for getting your projects moving faster.
Let's go over why JSONPlaceholder is so useful:
- Makes work faster - You don't have to wait for a server to be ready to start working on your website.
- Makes testing easier - Since you get data that's already formatted, checking if things work right is simpler.
- Great for trying out ideas - You can see how your app might look and work without needing a full backend.
- Keeps things simple at first - You can focus on the website's look and feel before worrying about the server.
- Straightforward to use - When it's time to switch to a real server, it's pretty easy because the setup is similar.
If you're ready to learn more and get better:
- Try keeping data around between sessions with local storage or files.
- Get comfortable with making API calls that wait for responses using async/await.
- Understand how to add, get, change, and remove data with CRUD operations.
- Dive into the documentation to learn about more things you can do with JSONPlaceholder.
- Set up your own server for testing with json-server if you want more control.
So, give JSONPlaceholder a go and see how it can make building websites a bit easier. It's a great way to speed up your work and learn more about how websites talk to servers. Have fun coding!
Related Questions
What is JSON placeholder API?
JSON Placeholder API is a free tool for developers. It gives you fake data to use when you're building and testing apps. This tool has pretend info like posts, comments, and user profiles that you can use.
How to make an API with JSON?
To make a simple API using JSON, follow these steps:
- First, make sure you have Node.js installed.
- Create a new folder for your project.
- Open your command line in this folder, and run
npm init
. - Use
npm install express
to add Express, which helps handle requests. - Make a
server.js
file where you'll write your code. - Use Express in your code to set up how your API responds.
- Add routes that send back JSON data when they're visited.
- Use
node server.js
to start your API.
You'll have routes in your API that send back data in JSON format.
What is difference between API and JSON?
- An API is like a messenger that takes requests and brings back responses between a computer or app and the internet. JSON is a way of writing data that's easy for both humans and computers to understand.
- APIs are about sending and getting data. JSON is about how that data is written.
- When you use an API, it might send data back written in JSON because it's easy to work with.
How to install JSON server using npm?
To set up JSON Server on your computer, just type this into your command line:
npm install -g json-server
This command installs JSON Server so you can use it to create a fake API based on a JSON file.