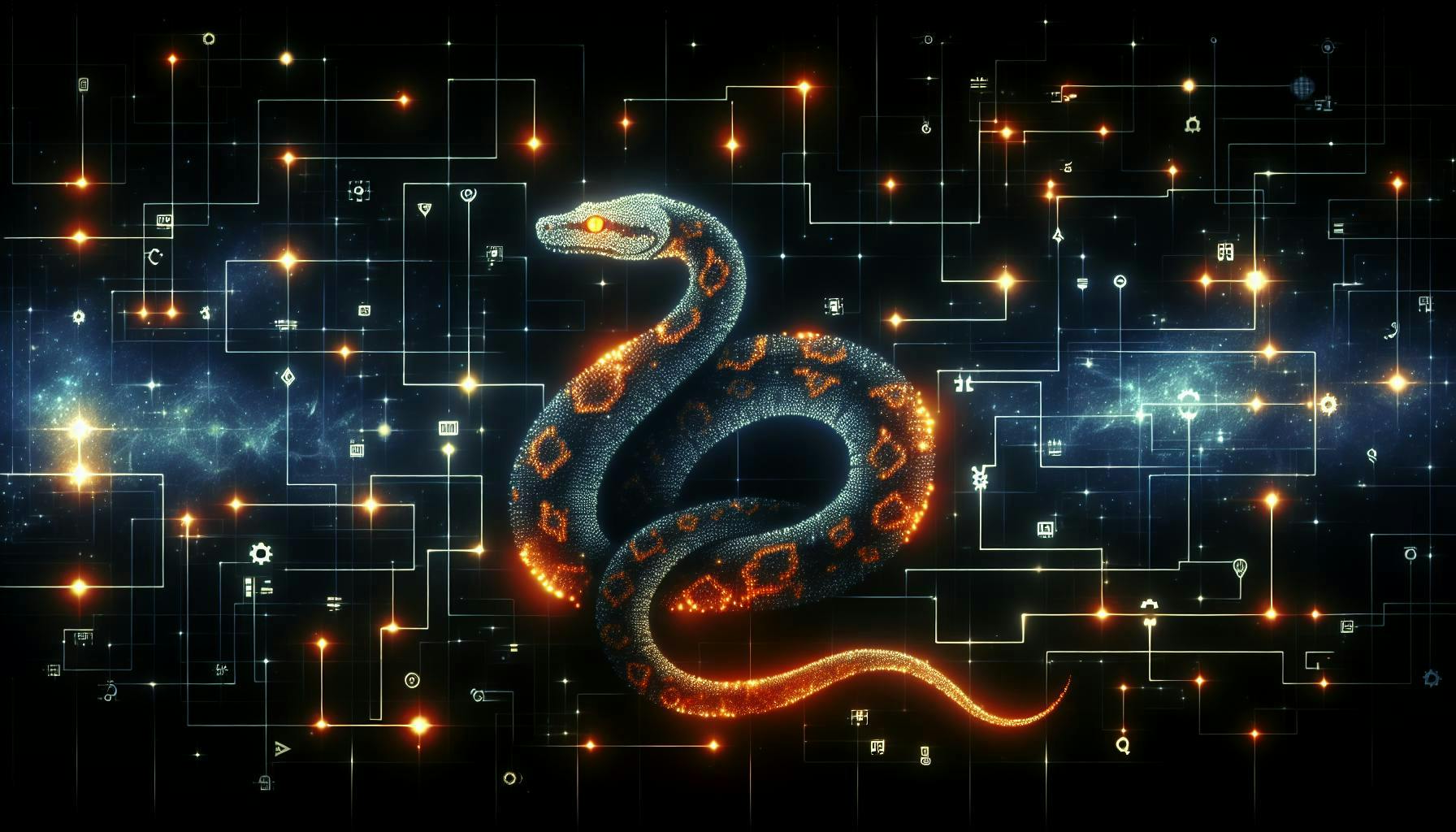
Swagger Python Django: Simplifying API Docs
Developing APIs can be tricky, especially when it comes to documentation. Most Django developers would agree that keeping API docs updated is a pain.
Luckily, there's a great tool that makes creating and maintaining API documentation much easier - Swagger. By integrating Swagger into your Django project, you can automatically generate beautiful API docs that always stay in sync with your code.
In this post, you'll learn how Swagger and the OpenAPI specification work, see examples of Swagger docs in action, and get step-by-step guidance on integrating Swagger into your Django REST Framework API to simplify documentation.
Introduction to Swagger and Django REST Framework API Documentation
Understanding Swagger and the OpenAPI Specification
Swagger is an open source framework for designing, building, documenting, and visualizing RESTful web services. It relies on the OpenAPI Specification (formerly Swagger Specification) to describe API structure and behavior in a language-agnostic interface description format.
Some key benefits of using Swagger include:
- Auto-generating interactive API documentation
- Providing a UI to easily test APIs
- Generating client SDKs for different languages
- Validating input and output payloads
Overall, Swagger simplifies API development across the entire lifecycle from design to deployment.
The Importance of API Documentation in Web Development
Comprehensive and accurate API documentation is critical for:
- Onboarding new developers
- Reducing support tickets and questions
- Speeding up integration and adoption
- Enabling productive API consumption
- Driving API usage and traffic
Without proper docs, developers struggle to understand an API's capabilities, endpoints, request/response formats, authentication methods, error codes, etc. Great API documentation vastly improves developer experience.
Integrating Swagger with Django for API Documentation
There are a few options for adding Swagger to Django projects:
- Django REST Swagger - Integrates the Swagger UI into Django REST Framework
- django-rest-swagger - Provides Swagger documentation for Django REST Framework APIs
- Drf-yasg - Swagger generator integrated with Django REST Framework
These tools auto-generate Swagger documentation from Django REST Framework code with customization options available.
Leveraging Django REST Framework with Swagger
Django REST Framework is a powerful toolkit for building Web APIs. Integrating Swagger brings additional benefits:
- Auto-generated interactive docs for Django REST APIs
- API explorer for testing endpoints
- Validation of requests/responses against schemas
- Customization of docs look and feel
- Enhanced development workflows
Together, Django REST Framework and Swagger offer a streamlined way to build, document, test and publish APIs.
Can I use Swagger with Django?
Swagger is a great tool for creating and documenting APIs with Django. Here's a quick overview of how you can integrate Swagger into your Django projects:
Use Django REST Framework
The easiest way to add Swagger to Django is by using the Django REST Framework (DRF). DRF has native support for generating OpenAPI schemas and Swagger UI.
To enable Swagger UI in DRF:
- Add
rest_framework
anddrf-yasg
to your Django project - Configure Swagger settings in your DRF settings file
- Add Swagger UI and schema endpoints in your project URLs
Now you'll have interactive API docs generated automatically from your DRF code!
Manual OpenAPI schema
You can also manually create an OpenAPI schema file and configure Swagger UI to use it. This involves:
- Creating a
openapi.yaml
file with your API schema - Setting up Swagger UI to point to your OpenAPI file
- Customizing Swagger UI as needed
This method takes more effort but allows full control over the API schema.
Other Django Swagger libraries
There are also other third-party libraries like django-rest-swagger and django-swagger-ui that can help integrate Swagger UIs into Django.
So in summary - yes, Swagger and Django work very well together! Leveraging DRF makes it easy, but you have options if you want more customization.
What is the difference between Django rest framework and Swagger?
Django REST framework (DRF) is a powerful toolkit for building Web APIs in Django. It makes it easy to create RESTful APIs that handle authentication, serialization, validation, throttling, and more.
Swagger UI, on the other hand, is a collection of static assets that can generate interactive documentation for APIs built with OpenAPI (formerly Swagger) specification. It provides a visual interface to make API requests and see responses.
So in summary:
- Django REST framework is a backend framework for creating REST APIs with Django
- Swagger UI is a frontend tool for visually documenting and testing APIs
Some key differences:
- DRF handles the server-side logic and infrastructure for building production-ready APIs
- Swagger UI generates interactive documentation for APIs, focused on the client-side dev experience
They can be used together to create well-structured APIs with great documentation:
- Use DRF to build out the API functionality
- Integrate swagger UI docs to allow easy testing/understanding of the API
This makes for a streamlined workflow - DRF handles all the complex backend work so developers can focus on business logic. And Swagger UI makes it simple for other devs to onboard and work with the APIs.
What is Swagger in Python?
Swagger is an open source set of tools that helps developers design, build, document and consume RESTful web services. It allows you to describe the structure of your APIs in a language-agnostic format, enabling both humans and machines to understand what the API does without having access to the source code.
Some key things that Swagger brings to the table for Python developers:
- API Documentation - Swagger auto-generates API docs from your code, including detailed information on routes, parameters, responses etc. This saves tons of time over manually creating documentation.
- Code Generation - Swagger can generate client libraries and server stubs for your API in various languages and frameworks including Python and Django. This accelerates app development.
- Testing - The Swagger UI allows developers to visually send test requests to an API without having any client code. This makes API testing and exploration much easier.
- Adoption - Swagger is extremely popular in the API community and has become something of an industry standard. Building Swagger APIs makes adoption simpler.
So in summary, Swagger enables Python developers to design, prototype, document and test REST APIs faster while also making it easier for others to understand and consume those APIs. The rich tooling cuts out many repetitive coding and documentation tasks.
sbb-itb-b2281d3
Is Django suitable for REST API?
Django can be a great choice for building REST APIs, especially if you need to integrate the API with other parts of a web application. Here are some key points on using Django for REST APIs:
Pros of using Django for REST APIs
- Django has built-in features that make building APIs easier, like serialization. This allows you to easily render Django models as JSON.
- There are several well-supported 3rd party packages like Django REST Framework that make building REST APIs faster. These handle things like requests, responses, serialization, and authentication.
- Since Django is full-stack, it's easy to share things like the database, models, authentication, etc. between a web frontend and API backend. This makes integration simpler.
- Django has a robust admin interface out of the box, which is useful for managing API resources and data.
- There is a strong community and ecosystem around Django if you need help.
Cons of using Django for REST APIs
- Django provides a lot of functionality you may not need for a pure API. This can add unnecessary complexity.
- Performance can become a bottleneck at scale since Django provides so much out of the box. Other lightweight frameworks may be faster.
- Django's focus is on server-rendered web apps rather than modern SPAs communicating via APIs. It doesn't have client-side rendering.
So in summary, Django is a good framework to develop a RESTful API backend if you also want to build a full-stack web application with Python. But if your only focus is the API, other frameworks like Flask or FastAPI might be better suited.
Integrating Swagger UI with Django REST Framework
Django REST Framework (DRF) has become the de facto standard for building REST APIs with Django. However, documenting these APIs can be tedious. This is where Swagger comes in handy.
Swagger is an open source set of tools that helps generate interactive API documentation from annotations in your code. When integrated with DRF, Swagger can automatically generate beautiful API docs with descriptions, parameters, models, endpoints and test capability.
There are a few open source options for integrating Swagger UI docs in Django projects. Let's explore some of the most popular ones.
Django REST Framework and Django-Swagger UI Compatibility
Django REST Swagger is a handy package that wraps Swagger UI around your DRF APIs.
To set it up:
- Install the package with
pip install django-rest-swagger
- Add
rest_framework_swagger
to your INSTALLED_APPS - Include the swagger URLs in your main urls.py:
urlpatterns = [
...
url(r'^docs/', include('rest_framework_swagger.urls'))
]
That's it! Django REST Swagger will now expose a /docs/
endpoint with an interactive Swagger UI for your API.
While simple to setup, django-rest-swagger has some limitations:
- Not designed for DRF 3.7+ projects
- Limited customization options
- No schema validation
For newer DRF APIs, other Swagger integration tools are recommended.
Automating Schema Generation with DRF-YASG
drf-yasg improves upon django-rest-swagger by:
- Officially supporting latest DRF 3.7+
- Flexible customization of UI
- Auto-generating OpenAPI 3.0 JSON schema
- Schema validation for correctness
To install:
pip install drf-yasg
And include it in INSTALLED_APPS:
INSTALLED_APPS = [
...
'drf_yasg',
]
drf-yasg scans your API views and serializers to automatically build a live Swagger UI and schema. This saves tons of manual documentation effort.
You can tweak the UI styles, branding, auth endpoints and more via Swagger settings. Drf-yasg also lets you override parts of the auto-generated schema when needed.
Overall, drf-yasg is the go-to choice for integrating Swagger with modern DRF codebases.
Enhancing API Documentation with Drf-spectacular
drf-spectacular is another excellent package for adding Swagger/OpenAPI 3.0 capabilities to Django REST Framework.
It focuses more on:
- Strict schema validation - ensures your API endpoints match what's documented
- Extensive customization - tailor the docs to your branding
- Enhanced descriptions - add more context to models, parameters, etc
- Multilingual docs - translate the UI into multiple languages
The schema auto-generation requires more explicit tagging versus drf-yasg. But you gain finer control over the API specifications.
For projects that demand strict validation and customization, drf-spectacular is a good choice for integrating Swagger.
Creating Swagger Documentation for a Django eCommerce API
Swagger is a useful tool for generating interactive documentation for REST APIs. In this section, we'll walk through configuring Swagger UI and OpenAPI schemas for a sample Django ecommerce API.
Overview of the Django eCommerce API Example
We'll be documenting a basic ecommerce API built with Django REST Framework. It has endpoints for:
- Browsing products
- Managing carts
- Placing orders
- User authentication
The API uses token authentication and provides JSON responses.
Setting Up Django-Swagger UI for Your API
To integrate Swagger UI with our Django API, we'll use the django-rest-swagger package:
- Install via pip:
pip install django-rest-swagger
- Add
rest_framework_swagger
to INSTALLED_APPS - Include the swagger URLs in urls.py
That's the basic setup. We can now access the Swagger UI at /swagger/
Customizing the OpenAPI Schema
The auto-generated OpenAPI schema contains the key information needed to power the Swagger UI. We can customize parts of it by creating a schema_view
that points to a custom SchemaGenerator
.
Some customizations we'll make:
- Descriptions for models and endpoints
- Tags and auth details
- Example bodies for POST/PUT requests
This helps provide clearer API documentation.
Integrating and Customizing the Swagger UI Docs Page
To make the Swagger UI more easily accessible, we'll create a route and view to serve the Swagger UI at /docs/
:
from rest_framework_swagger.views import get_swagger_view
schema_view = get_swagger_view(title='Ecommerce API')
urlpatterns = [
path('docs/', schema_view),
# ... other endpoints
]
We can also apply custom CSS and JS to brand the Swagger UI with the look and feel of our API documentation.
This ties the interactive docs directly into our existing API, completing the integration.
Best Practices for Maintaining High-Quality Django REST API Documentation
Maintaining comprehensive, up-to-date API documentation is critical for providing a great developer experience with your Django REST API. Here are some best practices for keeping your Swagger docs accurate, helpful, and synchronized with API changes.
Crafting Clear and Helpful API Descriptions
When writing OpenAPI schema descriptions for your Django REST API endpoints, parameters, and responses, consider these guidelines:
- Be concise yet descriptive: Summarize the purpose and functionality in 1-2 sentences. Provide relevant details without excessive verbosity.
- Explain parameter requirements: Note required formats, accepted values, etc. to prevent errors.
- Clarify response structures: Document the data structures returned, including field meanings.
- Use consistent voice and terminology: Maintain a consistent tone and vocabulary throughout for clarity.
For example, an effective endpoint description would be:
Returns a list of products. Accepts optional filtering parameters to narrow results by category, price range, etc. Responds with an array of product objects containing fields like ID, name, description, price.
Ensuring Your Swagger API Docs Stay Updated
Keeping your live Swagger documentation in sync with API changes is critical. Here's how to streamline the process:
- Integrate documentation into development workflow: Require developers to update docs as part of the pull request process. Perform doc review along with code review.
- Automate documentation builds: Configure your CI/CD pipeline to rebuild docs on each code deployment. This ensures docs reflect the live API.
- Use doc generation tools: Tools like drf-spectacular can auto-generate OpenAPI schema files from Django views and serializers. This reduces manual documentation work.
Regularly manually test your live Swagger UI with the API to catch any inaccuracies.
Testing Your Swagger Documentation for Accuracy
To validate the accuracy of your Swagger docs:
- Test endpoints manually: Exercise endpoints in the live API while cross-checking against Swagger UI, verifying input parameters, responses etc.
- Use reference values: Pass known valid/invalid inputs to confirm Swagger UI documented handling.
- Check error messaging: Intentionally trigger errors to verify accuracy of HTTP codes and error descriptions.
- Review complex structures: Ensure multi-level JSON responses match the defined output schema.
Fix any issues in the source OpenAPI files or docstrings to correct Swagger UI. Thorough testing ensures your API documentation remains a reliable reference!
Conclusion: Embracing Swagger for Effective Django REST Framework Documentation
Recap: The Advantages of Swagger in Django API Documentation
Swagger brings several key benefits when documenting Django REST Framework APIs:
- Auto-generated documentation based on Django views and serializers saves significant time over manually writing API docs.
- Documentation stays up-to-date with code changes since it's auto-generated. No need to manually update.
- Interactive documentation allows testing APIs right from the browser with the Swagger UI.
- Supports OpenAPI standard for defining REST APIs. Useful for integrating with other tools.
- Improves productivity by reducing time spent on manually creating and maintaining API docs. Teams can focus more on building features.
Overall, Swagger streamlines creating, visualizing and consuming REST API documentation in Django projects.
Exploring Further: Advanced Swagger Features for Django Developers
Beyond auto-generated API docs, Swagger offers other powerful capabilities:
- Code generation from OpenAPI definitions allows quickly scaffolding server stubs and client SDKs.
- API mocking helps front-end/mobile developers build out UI/apps before APIs are ready.
- Testing automation through Swagger definitions improves test coverage for REST APIs.
Learning these more advanced Swagger features allows taking productivity with Django REST APIs to the next level. Check out the Swagger open source tools and SwaggerHub for implementing them.